Leveling up with the F# Mentorship Program
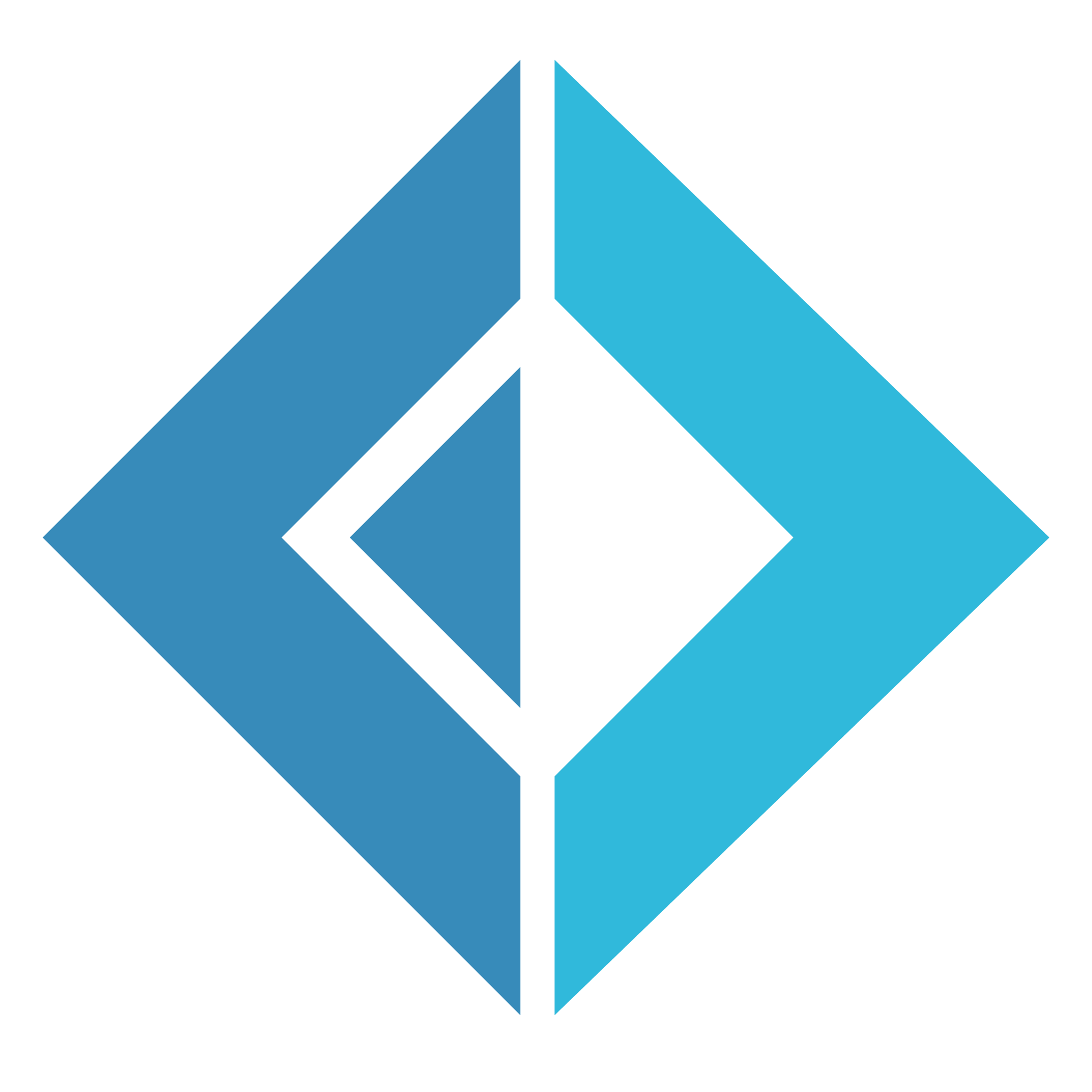
The more I code, the more I seem to run into functional programming. It started with wanting to write better JavaScript, then it grew to include wanting to write better Rust. My interest led me to start reading Structure and Interpretation of Computer Programs (SICP), and learning Scheme to go along with it. Now, I'm beginning a new phase with F#.
My theory was that if I could learn functional paradigms, I would be a better programmer, especially when it came to Rust. So I started researching Rust's influences. I heard Rust pulled a lot from the ML family of languages so I ran a search for ML. Ocaml was the first thing that popped up in my search so I started there. As it turns out, Facebook has been using a decent amount of Ocaml recently, and Reason ML - Facebook's new language for building typesafe JavaScript apps - is just a new syntax and toolchain for Ocaml. I found Ocaml and Reason to be unexpectedly beautiful languages. Languages I could really learn to love. I was about to dive into Reason ML when I discovered F#. A language very similar to Ocaml, but for .NET.
I've been interested in .NET for a while, but never had a reason to jump in. F# gave me that reason. It's one of the functional first languages I've been looking for, and it can leverage the .NET ecosystem - two birds with one stone. Also, it has first class support for Azure, and with Fable - an F# to JavaScript compiler - you can make JavaScript web apps as well. I'd see enough to know F# was the language for me, so I jumped on the F# Software Foundations website to poke around.
The F# Foundation website has tons of resources, but one thing in particular caught my eye. The F# Mentorship Program. I believe mentorship/apprenticeship can provide more opportunity for growth than any other sort of education, so I was naturally drawn to it. But at first I was hesitant. I assumed you either had to pay a decent amount to apply, or be a more senior developer than I am. It turns out that it's open to anyone, free of charge. Beginners, experts, open source contributors, anyone can apply! So I applied as soon as a new round opened up.
It was hard to believe at first, but I got a mentor! We meet up once a week for an hour or two over Skype, and the program lasts for six weeks. In our first week we went over some of the basics of F#, functional programming, and .NET by going through a tutorial called FSharp Koans. FSharp Koans leads you through the basics of F# via a series of tests. At the beginning, you're presented with a suite of failing tests, and your mission (should you choose to accept it), is to update the code, one section at a time, to pass each test. Once complete, you'll emerge an enlightened individual, ready to take on the world of functional programming.
If I wasn't already in love with the language, I definitely am now. A few things that really stuck out to me after my first week were the following:
-
F# has an amazing scripting environment called FSharp Interactive (FSI). I absolutely love this. It makes testing little snippets of code so easy and painless.
-
Functions are much more powerful than in a language like Java, but one of the coolest things is partial application. Partial application and currying is worth an entire blog post on its own, so here is a fantastic post on currying in F#. It's not the most exciting subject in the world, but it adds a lot of power and flexibility.
In JavaScript you can do some pretty cool stuff with closures. Take this adder function for example:
function adder(x) {
return function(y) {
return x + y;
}
}
let addTen = adder(10);
console.log(addTen(20)); //30
console.log(addTen(50)); //60
Using a closure we've created an addTen function to add 10 to any number we pass into it. It's a simple and silly example, but hopefully it gets the point across. But what if we just want to add two numbers in a single function call?
let getSum = adder(10, 20);
console.log(getSum); //getSum is a function, not 30
We can't use adder to add two values in a single function call. It's not a big deal, but wouldn't it be cool if we could?(Update: this is incorrect! You can add the two numbers with a "single" function call. Instead of adder(10, 20)
we would use adder(10)(20)
which would return 30
!) Lets see what my new friend F# can do:
let adder x y =
x + y
printfn "%d" (adder 10 10) //prints 20
let addTen = adder 10
printfn "%d" (addTen 30) //prints 40
With one function, we were able to create both behaviors, the closure version, and the one stop shop version. This is all accomplished thanks to currying!
- Pattern matching is awesome and beautiful:
//F#
let tellMeWhatItIs =
match x with
| 1 -> "it's a one"
| 2 -> "it's a two"
| _ -> "it's not one or two"
//JavaScript
let tellMeWhatItIs;
if (x == 1) {
tellMeWhatItIs = "it's a one";
} else if (x == 2) {
tellMeWhatItIs = "it's a two";
} else {
tellMeWhatItIs = "it's not one or two"
}
- Saving the best for last, when you join the F# foundation, you get access to the F# Slack Channel. Much like the Rust community, the F# community is full of some of unbelievably helpful people. Every channel is full of passionate people, ready to help fellow FSharpers at the drop of a hat.
Reference:
F# Software Foundation, Tour of F#, Fable, .NET, FSharp Koans